This guide contains PHP snippets. You should either add the snippets to your theme’s functions.php file or use the code snippets plugin (recommended).
Noptin does not come with a drag-and-drop email builder. Instead, it uses email templates to customize the look of your emails.
You can think of an email template as a WordPress theme. It is given the email heading, content, and footer text and then produces the final HTML email.
This allows you to do awesome things in your templates, such as using dynamic PHP code to generate truly personalized email content.
Noptin comes with several email templates.
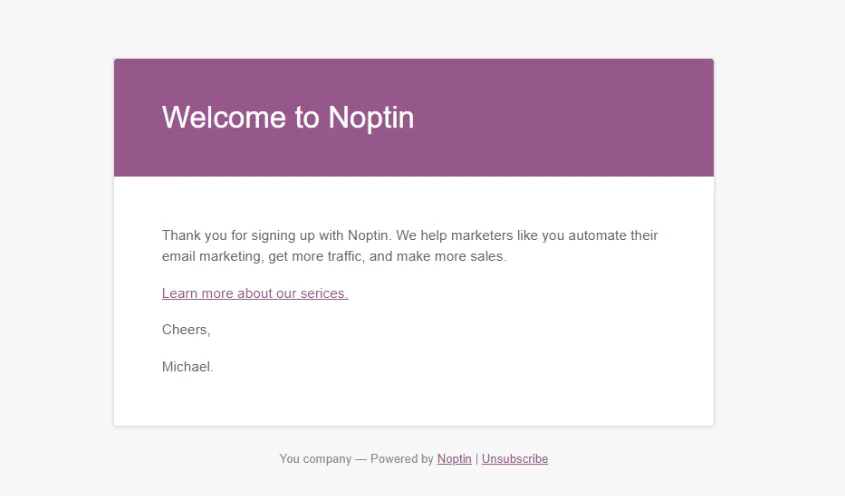
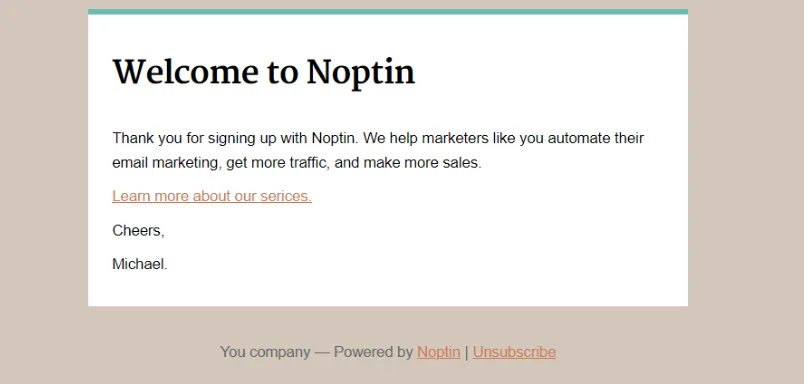
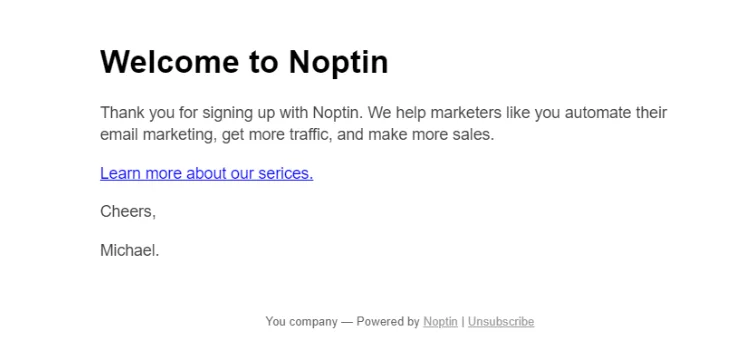
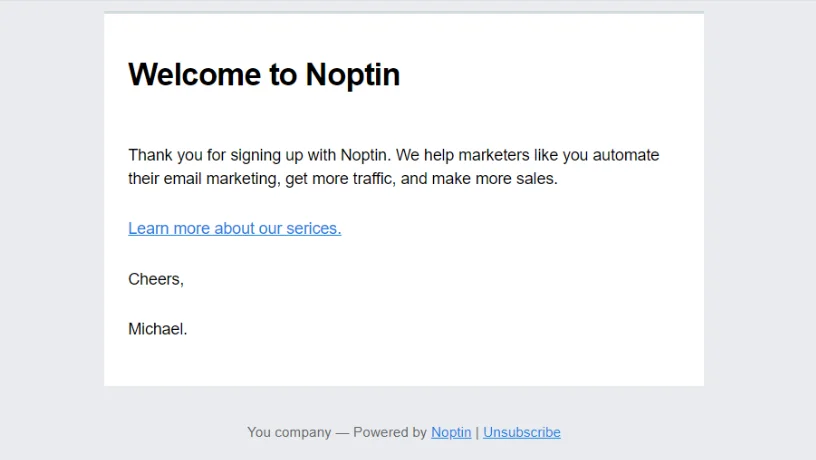
Changing an email’s template
You can use a different template for each email that you send with Noptin. This applies to both newsletter emails and automated emails.
To change your email template, open your email campaign in the Noptin email editor then scroll to the “Template” settings area and select your preferred template.
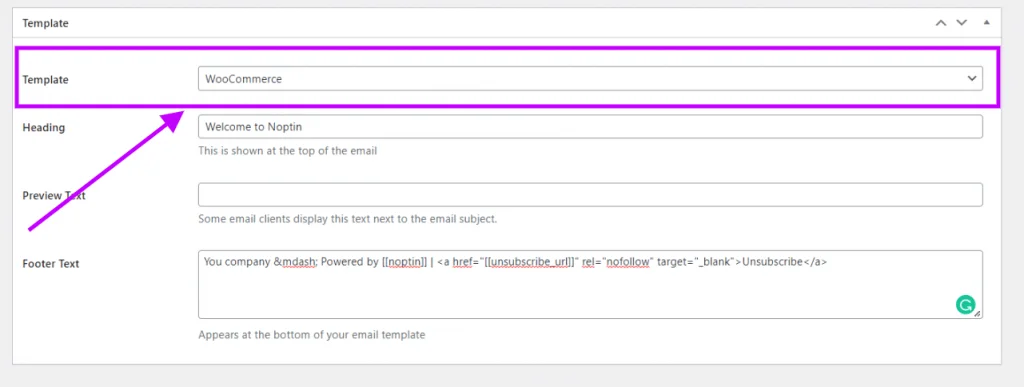
The “Template” settings area only appears if your email type is set to “Standard”.
Adding custom CSS to your email templates
You can use the following PHP snippet to add custom CSS to your emails.
<?php
add_action( 'noptin_email_styles', function() {
?>
a {
color: #008000;
}
<?php
});
This CSS will be applied to any emails you send with Noptin regardless of the email template you use. If you only want to style specific templates, use the following PHP snippet instead.
<?php
add_action( 'noptin_email_styles', function( $generator ) {
if ( 'my_template' !== $generator->template ) {
return;
}
?>
a {
color: #008000;
}
<?php
});
Customizing the default email templates
Nopin allows you to change the appearance of any built-in email template.
To do this:-
First, create a new folder in your active WordPress theme’s (or child theme’s) root folder and name it “noptin” as shown below.

Next, open the new folder and create a new “email-templates” folder as shown below.

Finally, copy the template that you want to customize from “wp-content/plugins/newsletter-optin-box/templates/email-templates” into the folder you previously created and customize it as you see fit.
You can use a plugin such as this one to customize the appearance of the WooCommerce template.
Creating a custom email template
You can also create new email templates.
To do this:-
First, you need to register your template via the noptin_email_templates
filter as shown below.
<?php
/**
* Registers a custom email template.
*
*/
function register_custom_noptin_email_template( $templates ) {
$templates['my-template'] = 'Custom Template';
return $templates;
}
add_filter( 'noptin_email_templates', 'register_custom_noptin_email_template' );
Finally, you need to generate the final HTML when an email is sent using your new template.
<?php
/**
* Registers a custom email template.
*
* @param string $generated_email
* @param Noptin_Email_Generator $generator
* @return string
*/
function apply_custom_noptin_email_template( $generated_email, $generator ) {
// Ensure that this is our template.
if ( $generator->template === 'my-template' ) {
ob_start();
?>
<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=<?php bloginfo( 'charset' ); ?>" />
<meta http-equiv="x-ua-compatible" content="ie=edge">
<title><?php echo esc_html( $generator->heading ); ?></title>
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="robots" content="noindex, nofollow" />
<style>
a { color: blue; }
</style>
</head>
<body>
<!-- start hero -->
<h1 style="margin-bottom: 20px;"><?php echo esc_html( $generator->heading ); ?></h1>
<!-- end hero -->
<!-- Start content -->
<div style="margin-bottom: 20px;"><?php echo wp_kses_post( trim( $generator->content ) ); ?></div>
<!-- end content -->
<!-- Start footer -->
<div style="margin-bottom: 20px;"><?php echo wp_kses_post( $generator->footer_text ); ?></div>
<!-- end footer -->
</body>
</html>
<?php
$generated_email = ob_get_clean();
}
return $generated_email;
}
add_filter( 'noptin_email_after_apply_template', 'apply_custom_noptin_email_template', 10, 2 );
The above code snippet is written in PHP. You can add it to your site via the Code Snippets plugin or by copying it to your theme’s functions.php file.
Using an email template builder
If you’re not a developer, you can also use an HTML email customizer plugin such as this one to change the appearance of your emails.
All you have to do is install and configure the plugin. It will then intercept Noptin emails and apply your configured template.
If you decide to go this route, make sure you set the template of each campaign to “No Template” as shown below.
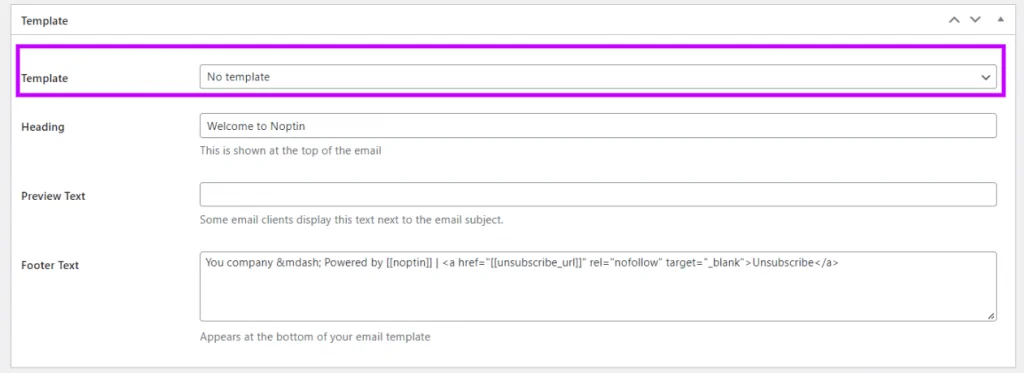
Using a drag and drop email builder
You can use any drag and drop email builder such as Unlayer to design your emails and send them via Noptin.
To do this:-
First, design the email in your email builder then export the email as an HTML document.
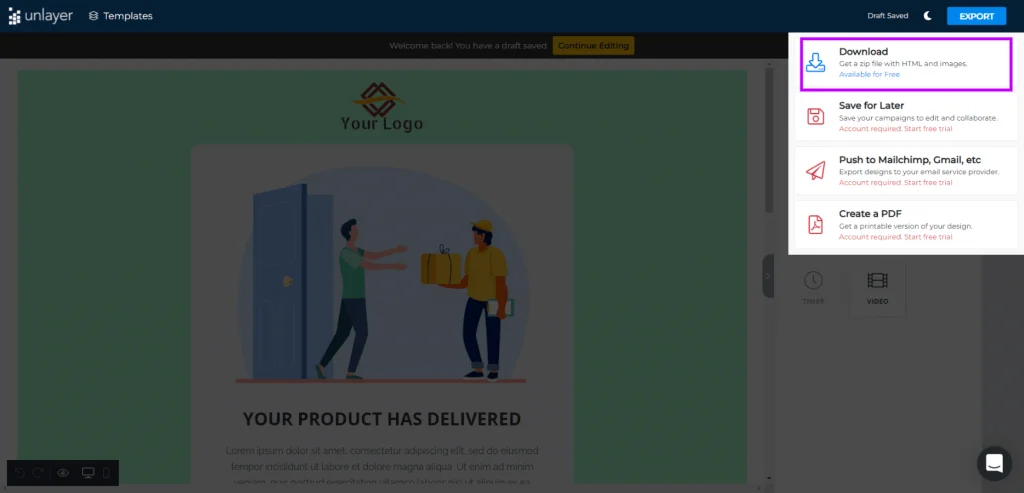
Second, create a new Noptin newsletter or automated email campaign then set the email type to “Raw HTML”.
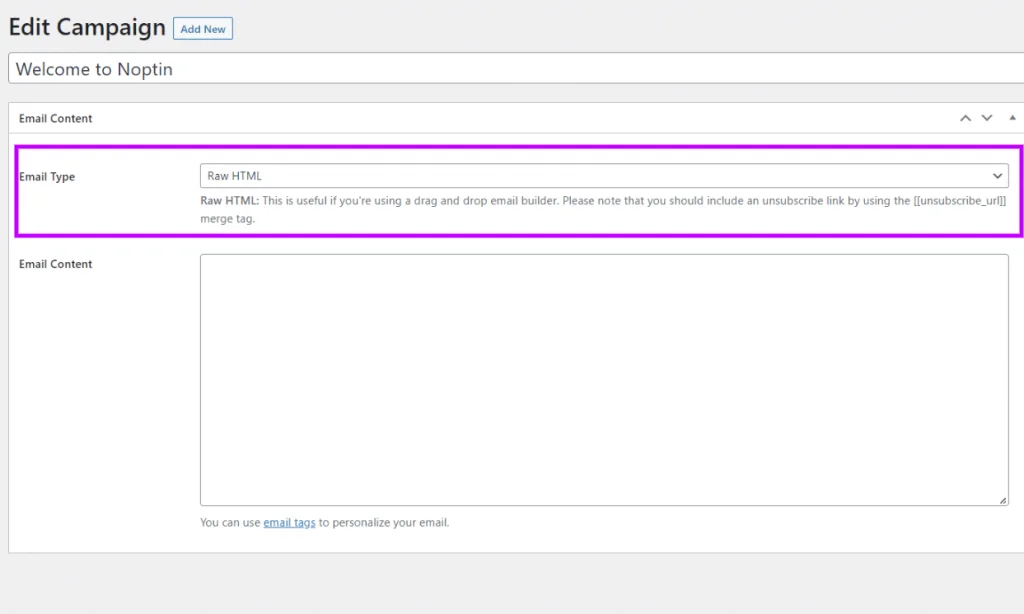
Finally, paste your exported HTML into the email content text area then either send or save your email campaign.
Remember to include an unsubscribe link in your email using the [[unsubscribe_url]] merge tag.
You can also personalize your email using any of our supported merge tags.
If your HTML email contains any images, remember to first upload the images to your site then edit the exported HTML and correct the image links.
Leave a Reply